Implement Mobile Websites Using the jQTouch Framework
Make your website mobile-device friendly
September 22, 2011
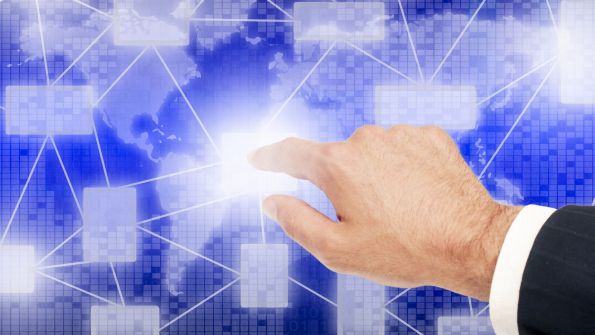
Mobile devices have become a necessity in today's tech-savvy world, and browsing mobile websites is a commonplace task. Unfortunately, most websites are not designed to be rendered on a mobile device, resulting in a bad experience for users. In this article, I will explain how to use the jQTouch framework to make websites compatible with all the popular mobile devices—and bring back the joy of web surfing to mobile-device users.
What Is jQTouch Framework?
jQTouch is a plug-in for the jQuery framework and provides native animations, automatic navigation, and themes for mobile WebKit browsers that run on devices such as iPhone, Android, and Palm Pre. The main purpose of the jQTouch framework is to make website navigation easier for users who are consuming the website on a mobile device.
The Scenario
For this article, we will work on a simple content-based web page and convert it to render it on a mobile device. For the sake of simplicity, we will focus on only a couple pages of the website, which comprises components such as Menu, Latest Articles, and Latest Videos. Figure 1 shows a screenshot of one of these pages, as it appears on a desktop browser. The web page does not illustrate styles or themes; such factors are not important for the premise of this article.

Figure 1: Demo web page
Designing for the Mobile Device
You might be tempted to use your existing web application design for mobile applications, but the results will not work as expected. Mobile applications should be designed from scratch and should include only the key functionality of the website. Keep in mind that the main purpose of designing a mobile website layout is to provide fast access to the site’s most used services.
Downloading the jQTouch Framework. The first step in using the jQTouch framework is to download it. You can easily download the jQTouch framework from the jQTouch website.
Activating the jQTouch Framework. The next step is to activate the jQTouch framework. To do so, add the necessary JavaScript and Cascading Style Sheets (CSS) folders to your project. Locate the jqtouch and themes folders inside your download folder, then copy these folders to your web application project:
jqtouch: This folder contains the required JavaScript and CSS files that are used by the jQTouch framework.
themes: This folder contains the CSS and images that are used to provide the theme for the display. The Apple theme is used by default, but you can change the theme any way you want.
After adding the folders to your project, add the necessary script and CSS references to your web page. Figure 2 shows the code for adding these references.
By adding the necessary styles and JavaScript files, we have enabled jQTouch for our web page. Now, we need to utilize those styles for the mobile version of the web page. jQTouch works by rendering predefined styles, creating the appearance of an application designed for the mobile device. Let's begin by defining the name of the toolbar. We do this by using the home class:
My website
Figure 3 shows how this code renders on the web page.

Figure 3: Toolbar added to the web page by using the jQTouch framework
Now, let's add an option to select the latest articles. Take a look at the following code:
My websiteLatest Articles
The ul tag allows us to add list items to the view. The edgetoedge class is responsible for stretching the list items all the way to the right of the screen. Figure 4 shows the effect of this code on the web page.

Figure 4: Latest Articles navigation link added to web page
The Latest Articles option will lead to other views, so it must be displayed by using an arrow sign. We can easily add an arrow to represent the hierarchical structure, by using the arrow class:
Latest Articles
Figure 5 shows the effect of this code on the web page.

Figure 5: Decorating ListItem with the CSS arrow class
Let's add another list item for Latest Videos and link both items to their corresponding subviews:
Latest ArticlesLatest Videos
At this point, we need to link the Latest Articles option with the Latest Articles view, in which all the most recent articles will be displayed. The linking mechanism works by using page jumps. The #latest_articles and #latest_videos links represent the IDs of each section that will be displayed when a link is clicked. The code in Figure 6 creates the latest_articles section.
Latest ArticlesArticle 1Latest VideosVideo 1
When a user clicks the Latest Articles link, the page loads the div element with the latest_articles ID, as Figure 7 shows.

Figure 7: Displaying static text when a navigation link is clicked
Implementing the Latest Articles View
Currently, the page displays the static text "Article 1" when a user clicks the Latest Articles link. The Latest Articles view is responsible for displaying the latest articles, so instead of displaying static text, we’ll make a web service that will return a JavaScript Object Notation (JSON)–formatted array. The web service is named ArticlesService and consists of the GetLatestArticles method, which Figure 8 shows.
[webMethod] public string GetLatestArticles() { var articles = new List() { new Article() { Title = "Introduction to jQTouch"}, new Article() { Title = "Designing for the Cloud"}, new Article() { Title = "Getting Started with NuGet"} }; // return articles; var json = new JavaScriptSerializer(); return json.Serialize(articles); }
GetLatestArticles is a straightforward method that creates a list of articles and then uses the JavaScriptSerializer class to return the JSON format to the client. Figure 9 shows the client-side code to invoke this web service method.
The request to the GetLatestArticles web method is fired when the user clicks the Latest Articles link, represented by the HTML anchor tag with the latest_articles ID. The click event triggers the $.ajax function, which makes the request. Inside the callback, we use the jquery-json library to parse the JSON into an object. Finally, we iterate through the articles object and append the new list items. Figure 10 shows the effect.Figure 10: List of latest articles displayedCreating the Article Detail ViewNext, we will build the final view, which will display the contents of the article on the screen. Again, we will be displaying the hard-coded data. When the user clicks a particular article title, an Ajax request is sent to the back end, which retrieves the details of the article and displays it in the view. For this process to work, we need to attach the onclick handler to each anchor tag that is used to display the articles. The onclick event invokes the GetArticleDetail function, passing articleId as the parameter:$("#ul_latest_articles").append("" + articles[i].Title + "");The getArticleDetail function is responsible for making the Ajax call to the web service and fetching the details of the article. Before looking at the implementation of the getArticleDetail function, we will take a look at the GetArticleDetail method on the web service: [webMethod] public string GetArticleDetail(int articleId) { var article = ArticleDataAccess.GetById(articleId); var json = new JavaScriptSerializer(); return json.Serialize(article); }ArticleDataAccess simply returns the dummy article instances, which are returned to the client side as a serialized JSON string. The getArticleDetail JavaScript function evaluates the returned JSON string and converts it to an object. Figure 11 shows the getArticleDetail function.function getArticleDetail(articleId) { var params = new Object(); params.articleId = articleId; $.ajax( { type: "POST", data: $.toJSON(params), dataType: "json", contentType: "application/json", url: "/ArticlesService.asmx/GetArticleDetail", success: function (response) { var article = $.evalJSON(response.d); $("#article_title").text(article.Title); $("#article_detail").append("" + article.Description + ""); } } ); }Figure 12 shows the effect of this code on the web page.Figure 12: Article detail viewRepresenting Page Sections as User ControlsTo keep the article simple, I put all the code in a single file. For larger applications, you can represent each section as a separate user control. This technique has several benefits, including enabling caching of particular sections and easily modification of sections without interfering with other parts of the page.Easy Navigation on Mobile DevicesMobile devices are becoming many users' first choice for consuming information. Websites must be designed specifically to render correctly on mobile devices. jQTouch allows us to create smart-phone–independent sites that provide easy navigation on these devices. jQTouch applications can also be deployed in the Apple App Store as iPhone applications—with the help of PhoneGap, which I plan to cover in an upcoming article.
About the Author(s)
You May Also Like