workers collaborate around computers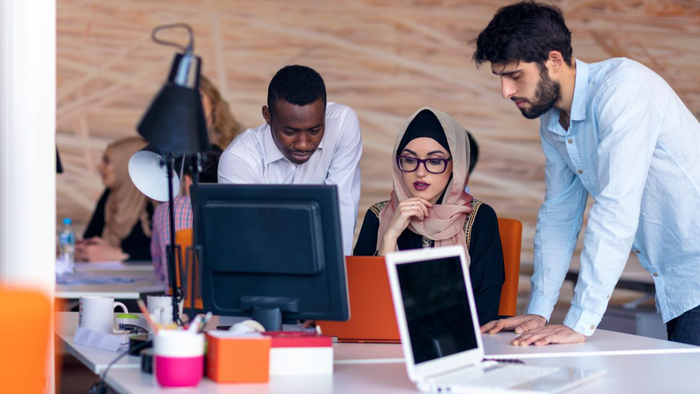
IT Management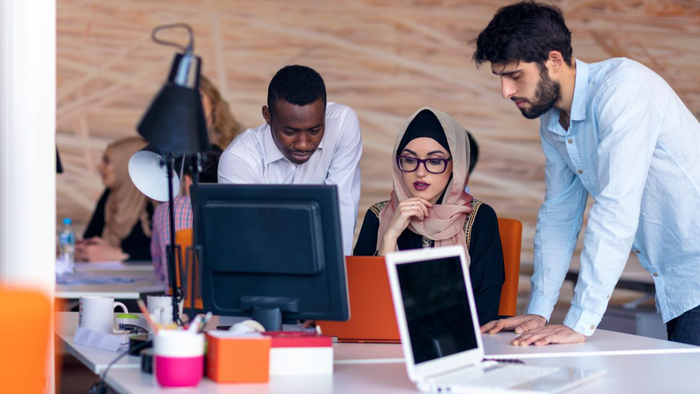
3 Actions to Create an IT Strategic Workforce Plan3 Actions to Create an IT Strategic Workforce Plan
In an era of rapid digital disruption, strategic workforce planning is crucial for aligning IT talent with business goals, addressing skills gaps, and ensuring organizational success.
Sign up for the ITPro Today newsletter
Stay on top of the IT universe with commentary, news analysis, how-to's, and tips delivered to your inbox daily.